Understanding the problem:
We are going to count the consecutive occurrences of the characters in a given string. The character may repeat multiple time with different consecutive counts. Characters count should not be case sensitive. Lets look at some of the examples.
Ex1: Input: aahnmkhhlkknjm
Output: a : 2, h : 1, n : 1, m : 1, k : 1, h : 2, l : 1, k : 2, n : 1, j : 1, m : 1
Ex2: Input: recommended
Output: r : 1, e : 1, c : 1, o : 1, m : 2, e : 1, n : 1, d : 1, e : 1, d : 1
Ex3: Input: recoMmended
Output: r : 1, e : 1, c : 1, o : 1, m : 2, e : 1, n : 1, d : 1, e : 1, d : 1
Approach:
First we'll have a set of variables to store the input string, an array of characters to store the input string, current character being counted, count of the occurrences., and a list to store the character and its consecutive count value.
First, we convert the given string to character array (We can use String.charAt(int) instead. You will find the example with both approaches below). first set current=chars[0] and count=1. Now we have considered first character. Lets start from the second character and start finding the consecutive occurrences. We start the for loop setting i=1, till i<chars.length. check whether the current is equals to chars[i]. If it equals, increment the count as these are consecutive characters. Otherwise, these are not consecutive characters, add current and count to the charCounts, set count to 1 and current to chars[i]. when i reaches chars.length, the loop terminates. Remember, current and count in last iteration are not added to the charCounts. Add it after loop completion. Now you have the desired output. We want the count to be case insensitive, use .toLowerCase() on string as shown in example. Lets ave a look at algorithm and flowchart below. Feel free to post your doubts or opinions in the comment section below.
Algorithm:
Step1: Start
Step2: Declare variables - string, chars, charCounts, current, count, c
Step3: Read input string.
Step4: Initialize - chars = char array of string, current = chars[0], count = 1 and charCounts = new list.
Step5: Repeat from i = 1 to chars length
Step5.1: Assign c = chars[i]
Step5.2: If current equals c
increment count
else
add current and count values to charCounts
reset count to 1.
set current = c
Step6: add current and count values to charCounts for last character.
Step7: Display charCounts
Step8: Stop
Flow Chart
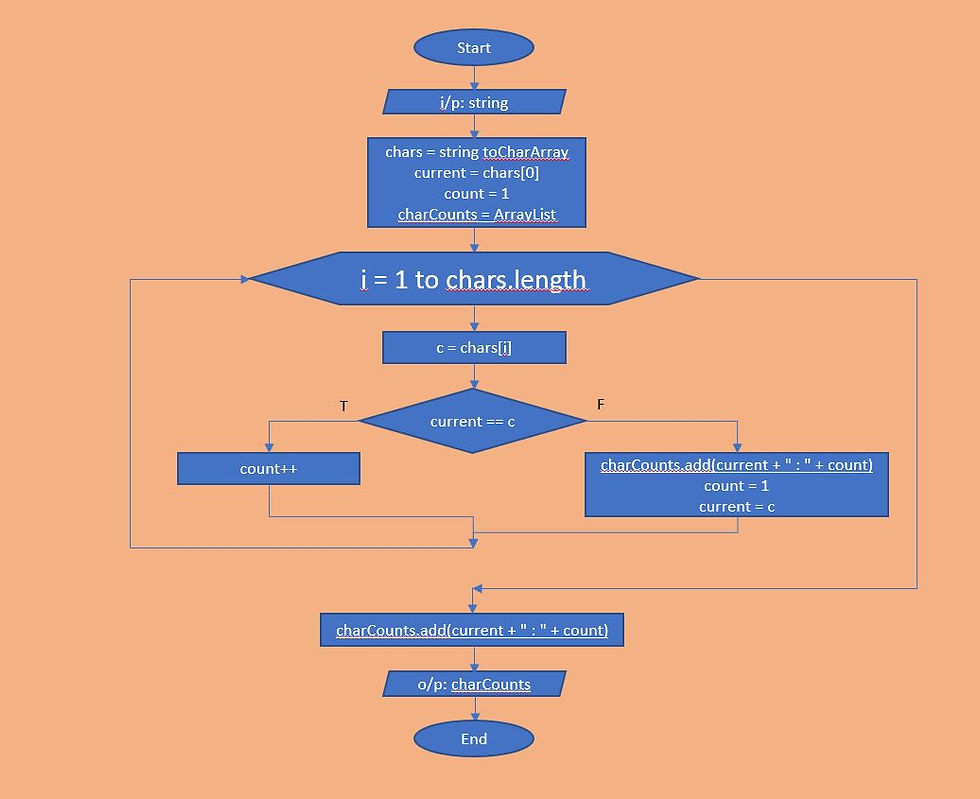
Java Code.
import java.util.ArrayList;
import java.util.Collection;
public class Sample {
public static void main(String[] args) {
String string = "recoMmendee";
System.out.println(getConsecutiveOccurrenceOfChar(string.toLowerCase()));
System.out.println(getConsecutiveOccurrenceOfChar(string.toLowerCase().toCharArray()));
}
// Example using char arrays
private static Collection<String> getConsecutiveOccurrenceOfChar(char[] chars) {
Collection<String> charCounts = new ArrayList<>();
char current = chars[0];
int count = 1;
for(int i = 1; i < chars.length; i++){
char c = chars[i];
if(current == c) {
count++;
} else {
charCounts.add(current + " : " + count);
count = 1;
current = c;
}
}
charCounts.add(current + " : " + count);
return charCounts;
}
// Example using .charAt(int)
private static Collection<String> getConsecutiveOccurrenceOfChar(String string) {
Collection<String> charCounts = new ArrayList<>();
char current = string.charAt(0);
int count = 1;
for(int i = 1; i < string.length(); i++){
char c = string.charAt(i);
if(current == c) {
count++;
} else {
charCounts.add(current + " : " + count);
count = 1;
current = c;
}
}
charCounts.add(current + " : " + count);
return charCounts;
}
}
Comentários