Insert Star(*) between consecutive even digits and Hyphen(-) between consecutive odd digits.
- Bhimubgm
- Feb 20, 2019
- 2 min read
Understanding the problem:
We are going to insert star(*) between any two consecutive even numbers and hyphen(-) between any two consecutive odd numbers. The digit zero(0) is not considered as either even or add. The give number in the form of string could be transformed like following examples.
Ex1: Input: "98234925928"
Output: 98*234925-92*8
Ex2: Input: "2397492834092"
Output: 23-9-7492*834092
Approach:
We are going to take input(numbers) as string. Assume that input is positive integer. Have a variable to store the formatted output. Lets start putting first character to output string. Start a loop from i = 0 till numbers.length. Now first we have to make sure the digit at index i or i+1 is not zero. We don't consider zero as even/odd digit as per our problem definition. We know that reminder after dividing an even digit by two is always zero. So, now check if both the digits at index i or i+1 are even applying modulus(%) operator. If both are even, add star(*) to output string. Else, check if both are even. If they are then add hyphen(-) to output string. Now add digit at index i+1 to output string. Once you are out of the loop, you have the desired output. Lets ave a look at flowchart below. Feel free to post your doubts or opinions in the comment section below.
Flowchart:
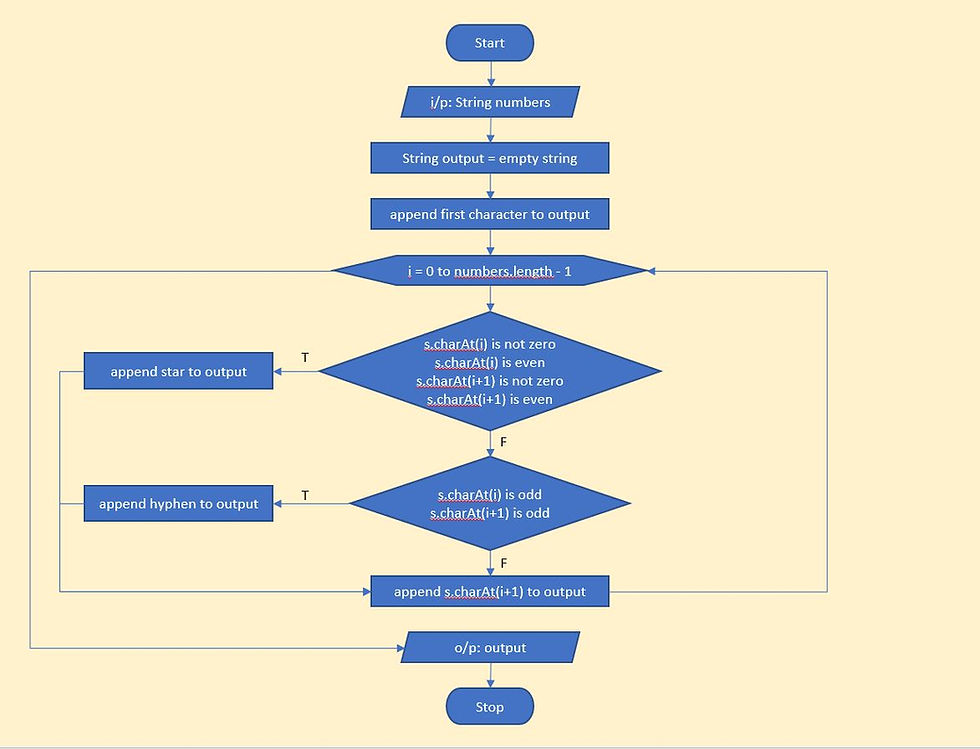
Java Code:
package com.bhimubgm.example;
public class InserStartAndHyphen {
public static void main(String[] args) {
System.out.println(inserStartAndHyphen("2397492834092"));
}
public static String inserStartAndHyphen(String s){
// Stores resulting string.
StringBuilder sb = new StringBuilder();
// Start with the first character
sb.append(s.charAt(0));
for(int i = 0; i < s.length() - 1; i++){
int v1 = Integer.parseInt("" + s.charAt(i));
int v2 = Integer.parseInt("" + s.charAt(i + 1));
// Check if both or even numbers then appen '*'
if(v1 != 0 && v2 != 0 && v1 % 2 == 0 && v2 % 2 == 0) {
sb.append('*');
}
// Check if both are odd numbers then append '-'
else if(v1 % 2 != 0 && v2 % 2 != 0){
sb.append('-');
}
//put next character in result.
sb.append(s.charAt(i + 1));
}
return sb.toString();
}
}
Comments