Find longest common suffix between two strings.
- Bhimubgm
- Feb 20, 2019
- 1 min read
Updated: Feb 22, 2019
Understanding the problem:
We are going to find the longest suffix between any two strings given. If no common suffix is found then output should be NULL. Check out following examples for better understanding.
Ex1: Input: "asfdsdfgrg" and "sdasdfgrg"
Output: sdfgrg
Ex2: Input: "tyhtgaerge" and "gfhjwertw"
Output: NULL
Approach:
Our program takes two input strings and computes the longest common suffix between these two. As we have to find suffix, we start from the end of the strings. Keep comparing the characters from the tail of the both strings moving towards the forth by one index each time. Every time we have match, increment the suffix length by one. Once we find non matching characters, we have found the longest common suffix, exit the loop. Lets make sure suffix length is greater than zero. If zero, no common suffix, output - NULL. Else, compute suffix using sub-string . At the end you have the desired output. Lets ave a look at flowchart below. Feel free to post your comments or opinions in the comment section below.
Flowchart:
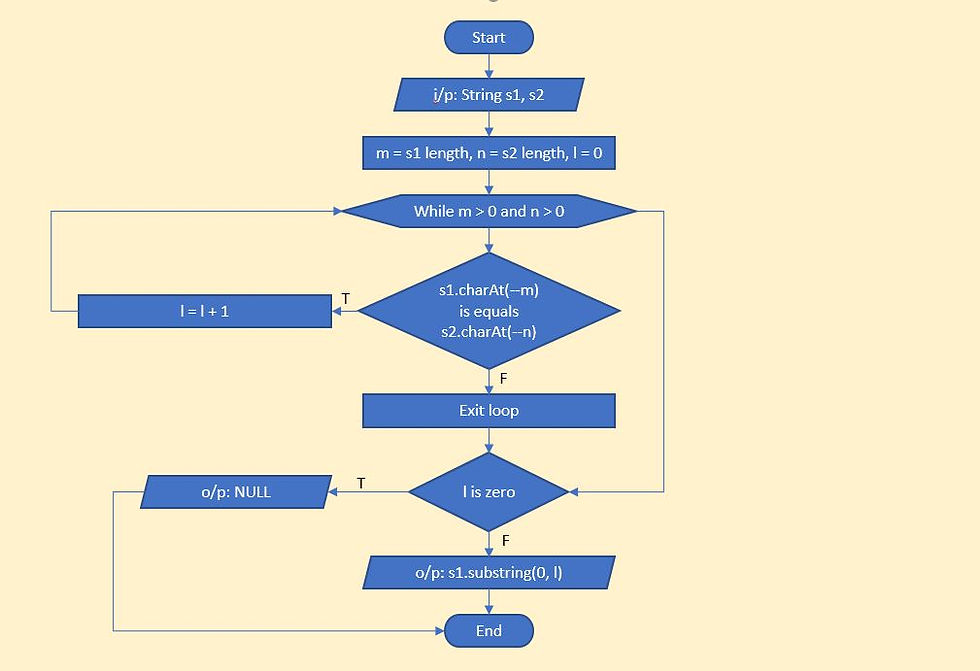
Java Code:
package com.bhimubgm.example;
public class LongestCommonSuffix {
public static void main(String[] args) {
System.out.println(findLongestCommonSuffix("asfdsdfgrg", "sdasdfgrg"));
}
public static String findLongestCommonSuffix(String s1, String s2) {
int m = s1.length();
int n = s2.length();
// Store length of longest suffix
int l = 0;
while(m > 0 && n > 0){
if(s1.charAt(--m) == s2.charAt(--n)){
l++;
} else {
break;
}
}
// There is no common suffix
if(l == 0){
return "NULL";
}
// Stores longest suffix
String longestSiffix = "";
while(l > 0){
longestSiffix += s1.charAt(++m);
l--;
}
return longestSiffix;
}
}
Comments